HTTP
This page has been automatically translated using the Google Translate API services. We are working on improving texts. Thank you for your understanding and patience.
Functions
Http* | http_create (...) |
Http* | http_secure (...) |
void | http_destroy (...) |
void | http_clear_headers (...) |
void | http_add_header (...) |
bool_t | http_get (...) |
bool_t | http_post (...) |
uint32_t | http_response_status (...) |
const char_t* | http_response_protocol (...) |
const char_t* | http_response_message (...) |
uint32_t | http_response_size (...) |
const char_t* | http_response_name (...) |
const char_t* | http_response_value (...) |
const char_t* | http_response_header (...) |
bool_t | http_response_body (...) |
Stream* | http_dget (...) |
bool_t | http_exists (...) |
It is common for an application to need information beyond that stored on the computer itself. The simplest and most common way to share information is to store it on a Web Server and publish a URL that provides the desired content (Figure 1). This client/server scheme uses the HTTP/HTTPS protocol, which was originally designed to transmit HTML documents between web servers and browsers. Due to the great impact it has had over the years, its use has been expanding for the exchange of structured information between any application that "understands" HTTP. The response from the server will usually be a block of text formatted in JSON or XML.
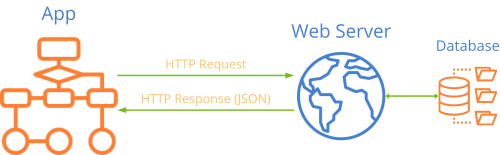
- Use http_dget to download a resource from its URL (Listing 1).
- Use http_create to create an HTTP session.
- Use http_secure to create an HTTPS session (encrypted).
1 2 3 4 5 6 7 8 9 |
On the other hand, if we are going to make successive calls to the same server or if we need more control over the HTTP headers, we must create a session (Listing 2).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
Stream *webpage = NULL; Http *http = http_secure("nappgui.com", UINT16_MAX); if (http_get(http, "/en/start/win_mac_linux.html", NULL, 0, NULL) == TRUE) { if (http_response_status(http) == 200) { webpage = stm_memory(1024); if (http_response_body(http, webpage, NULL) == FALSE) stm_close(&webpage); } } http_destroy(&http); if (webpage != NULL) { ... stm_close(&webpage); } |
http_create ()
Create an HTTP session.
Http* http_create(const char_t *host, const uint16_t port);
host | Server name. |
port | Connection port. If we pass |
Return
HTTP session.
http_secure ()
Create an HTTPS session.
Http* http_secure(const char_t *host, const uint16_t port);
host | Server name. |
port | Connection port. If we pass |
Return
HTTP session.
http_destroy ()
Destroy an HTTP object.
void http_destroy(Http **http);
http | The HTTP object. Will be set to |
http_clear_headers ()
Remove previously assigned HTTP headers.
void http_clear_headers(Http *http);
http | HTTP session. |
http_add_header ()
Add a header to the HTTP request.
void http_add_header(Http *http, const char_t *name, const char_t *value);
http | HTTP session. |
name | The name of the header. |
value | The header value. |
http_get ()
Make a GET request.
bool_t http_get(Http *http, const char_t *path, const byte_t *data, const uint32_t size, ierror_t *error);
http | HTTP session. |
path | Resource. |
data | Data to add in the body of the request. It can be |
size | Data block size in bytes. |
error | Error code if the function fails. It can be |
Return
TRUE
if the request has been processed correctly. If FALSE
, in error
we will have the cause.
Remarks
The request is synchronous, that is, the program will be stopped until the server responds. If we want an asynchronous model we will have to create a parallel thread that manages the request. HTTP redirections are resolved automatically.
http_post ()
Make a POST request.
bool_t http_post(Http *http, const char_t *path, const byte_t *data, const uint32_t size, ierror_t *error);
http | HTTP session. |
path | Resource. |
data | Data to add in the body of the request. It can be |
size | Data block size in bytes. |
error | Error code if the function fails. It can be |
Return
TRUE
if the request has been processed correctly. If FALSE
, in error
we will have the cause.
Remarks
See http_get.
http_response_status ()
Returns the response code of an HTTP request.
uint32_t http_response_status(const Http *http);
http | HTTP session. |
Return
The response code from the server.
http_response_protocol ()
Returns the protocol used by the HTTP server.
const char_t* http_response_protocol(const Http *http);
http | HTTP session. |
Return
The server protocol.
http_response_message ()
Returns the response message from the HTTP server.
const char_t* http_response_message(const Http *http);
http | HTTP session. |
Return
The response message from the server.
http_response_size ()
Returns the number of response headers from an HTTP request.
uint32_t http_response_size(const Http *http);
http | HTTP session. |
Return
The number of headers.
http_response_name ()
Returns the name of the response header of an HTTP request.
const char_t* http_response_name(const Http *http, const uint32_t index);
http | HTTP session. |
index | The index of the header (0, size-1). |
Return
The name of the header.
http_response_value ()
Returns the value of the response header of an HTTP request.
const char_t* http_response_value(const Http *http, const uint32_t index);
http | HTTP session. |
index | The index of the header (0, size-1). |
Return
The value of the header.
http_response_header ()
Returns the value of a response header from an HTTP request.
const char_t* http_response_header(const Http *http, const char_t *name);
http | HTTP session. |
name | The name of the desired header. |
Return
The value of the header. If the header does not exist, it will return an empty string ""
.
http_response_body ()
Returns the response body of an HTTP request.
bool_t http_response_body(const Http *http, Stream *body, ierror_t *error);
http | HTTP session. |
body | Write stream where the response content will be stored. |
error | Error code if the function fails. It can be |
Return
TRUE
if it was read successfully. If FALSE
, in error
we will have the cause.
http_dget ()
Make a direct request for a web resource.
Stream* http_dget(const char_t *url, uint32_t *result, ierror_t *error);
1 2 3 4 5 6 |
url | Resource URL. |
result | Server response code. It can be |
error | Error code if the function fails. It can be |
Return
Stream with the result of the request.
Remarks
Use this function for direct access to an isolated resource. If you need to make several requests or configure the headers, use http_create or http_secure.
http_exists ()
Check if a web resource is available / accessible.
bool_t http_exists(const char_t *url);
url | Resource URL. |
Return
TRUE
if the resource (web page, file, etc) is accessible.
Remarks
HTTP redirections are not resolved. It will return FALSE
if the URL as is is not valid.